1. introduction to Spring
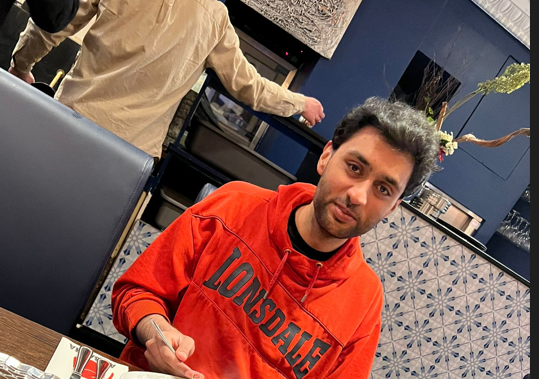
Haseeb Akbar
3 - mins
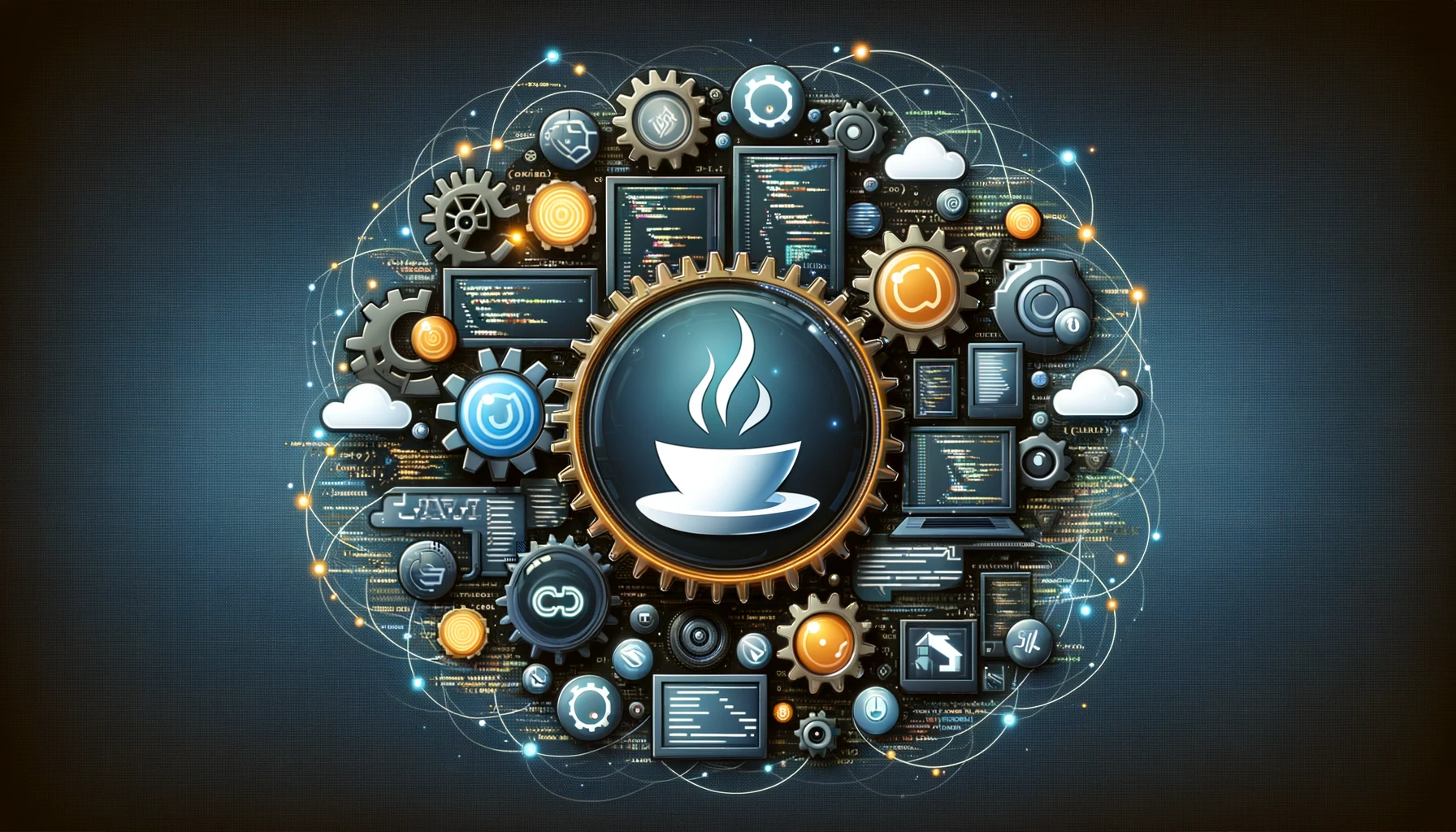
Coding
What is Spring?
- Spring Framework: A powerful, lightweight framework for building Java applications. It simplifies the development of enterprise-grade applications through support for various infrastructural concerns (e.g., transaction management, security, etc.).
Why is Spring Useful?
- Simplified Dependency Management: Manages libraries and dependencies in your project efficiently, reducing conflicts and compatibility issues.
- Promotes Good Practices: Encourages developers to follow best practices and design patterns, such as Dependency Injection (DI) and Aspect-Oriented Programming (AOP).
- Versatility: Supports a wide range of applications, from small stand-alone applications to large, complex enterprise systems.
Dependency Injection (DI)
Dependency Injection is a design pattern used to implement IoC (Inversion of Control), where the control over the dependencies of a class shifts from the class itself to a framework or container. In simpler terms, DI allows classes to be more loosely coupled, making them more modular, easier to test, and maintain.
How DI Works:
- Without DI: Typically, an object creates its own dependencies, leading to tight coupling between the components. Changing a dependency might require changes to the dependent class.
- With DI: Dependencies are "injected" into an object at runtime, rather than being hard-coded within the object. This can be done through constructors, setters, or properties.
Benefits of DI:
- Decoupling: Classes do not need to know about the creation and configuration of their dependencies, leading to a decoupled architecture.
- Testability: Easier to test classes by injecting mock dependencies, facilitating unit testing and behaviour testing without requiring the actual dependencies.
- Flexibility and Reusability: Components can be easily replaced or reused in different contexts since they are not tightly coupled to their dependencies.
Aspect-Oriented Programming (AOP)
Aspect-Oriented Programming is a programming paradigm that aims to increase modularity by allowing the separation of cross-cutting concerns. It does so by adding additional behaviour to existing code (an advice) without modifying the code itself.
Key Concepts:
- Aspect: A module that encapsulates behaviours that affect multiple classes into reusable modules. It's the AOP equivalent of a class in OOP.
- Join Point: A point during the execution of a program, such as the execution of a method or the handling of an exception.
- Advice: Action taken by an aspect at a particular join point. Different types of advice include "before," "after," and "around" that define when the code should be executed in relation to the join point.
- Pointcut: A predicate that matches join points. Advises are associated with a pointcut expression and run at any join point matched by the pointcut (for example, execution of methods with a certain annotation).
- Target Object: Object being advised by one or more aspects. It's often a proxy object in the Spring Framework, allowing it to weave in advice with the target object's method calls.
Benefits of AOP:
- Separation of Concerns: By separating cross-cutting concerns (like logging, transaction management, or security) from the business logic, the code becomes cleaner and more maintainable.
- Reduced Code Duplication: Common functionalities that are spread across multiple classes can be centralised in aspects, reducing code duplication.
- Improved Code Modularity: AOP makes it easier to add or change cross-cutting concerns without touching the core business logic, leading to more modular and adaptable code.
What is Spring Boot?
- Spring Boot: An extension of the Spring framework that simplifies the initial setup and development of new Spring applications. It offers a way to create stand-alone, production-grade Spring based applications easily.
Why is Spring Boot Useful?
- Simplified Dependency Management: Automates and manages dependencies effectively with the concept of starters (e.g.,
spring-boot-starter-web
for web applications), making dependency management manageable. - Simplified Deployment: Simplifies the deployment process by creating self-contained applications that don't require an external servlet container.
- Autoconfiguration: Automatically configures your application based on the libraries you have added, making it easier to get a project up and running quickly.
Three Core Features of Spring Boot
- Simplified Dependency Management:
- Uses a set of "Starters" (e.g.,
spring-boot-starter-web
) to bundle common dependencies together, simplifying the build configuration. - Leverages Bill of Materials (BOMs) to ensure that all dependencies work well together without version conflicts.
- Simplified Deployment:
- Creates self-contained applications that can be run as standalone Java applications, simplifying the deployment process.
- Removes the need for external application servers by embedding servers like Tomcat, Jetty, or Undertow directly.
- Autoconfiguration:
- Automatically configures your Spring application based on the jar dependencies you added. For example, if
spring-boot-starter-web
is in your class path, Spring Boot auto-configures a web application. - Described as "magic" by newcomers, it significantly reduces the need for manual configuration and boilerplate code.
Dependency Management Challenges
- Dependency Conflicts: Using multiple libraries together can lead to version conflicts, where one library's update breaks another.
- Complexity: Managing dependencies and ensuring compatibility across different environments can be complex and time-consuming.
Bill of Materials (BOMs)
- A BOM is a special kind of POM (Project Object Model) in Maven that defines a list of dependencies and their versions that are known to work well together.
- Spring Boot uses BOMs to control the versions of dependencies, making it easier to manage them without specifying versions explicitly.
Spring Boot Starter Web
- A starter that provides all the necessary dependencies to build a web application. This includes embedded servers, Spring MVC, and more, bundled in a single dependency.
Application Servers and Spring Boot
- Before Spring Boot: Deploying Java applications involved installing and configuring an application server, managing database drivers, creating database connections, and more.
- With Spring Boot: These steps are simplified or automated, making the development and deployment process much smoother.
Shading & Nesting Jars
- Shading: The process of creating a uber-jar (or fat jar) that contains all of your application's dependencies packaged together. This helps in avoiding conflicts with other jars by renaming packages.
- Nesting Jars: Spring Boot supports nesting jars (jars within a jar), which is part of how it creates executable jars. This makes deployment easier by packaging everything needed to run the application into a single executable jar.
Autoconfiguration: The "Magic"
- Spring Boot's autoconfiguration automatically sets up your application based on the dependencies you have added, reducing the need for explicit configuration and making it easier for beginners to start projects.